RS485
Hardware
One RS485/RS422 bus is integrated into the AutomatePro with a max data rate of 500kbs. A 120ohm termination resistor should be placed between RS485P and RS485N at both ends of the RS485 network.
Connector Pinout
- Connector
- Development Breakout Board
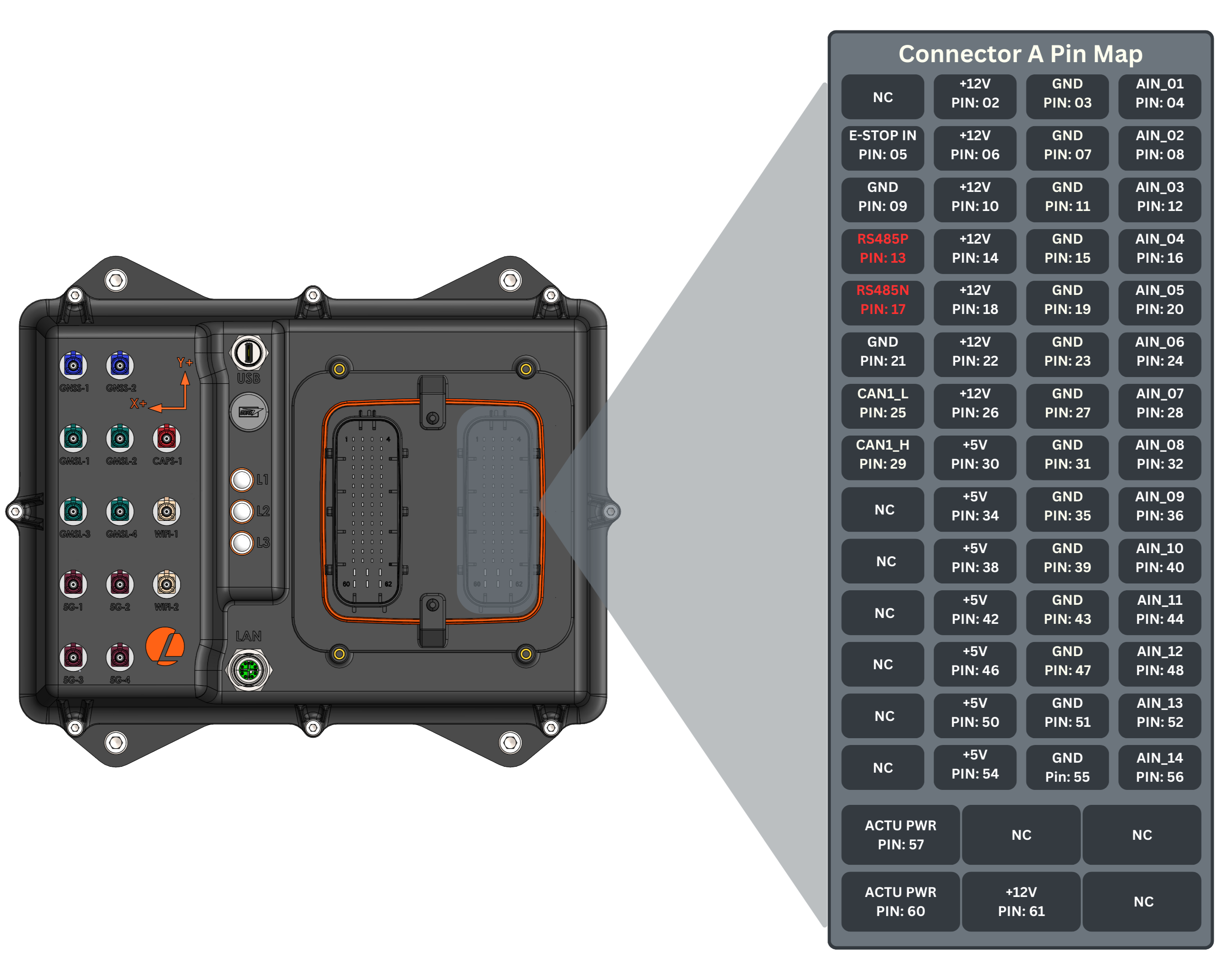
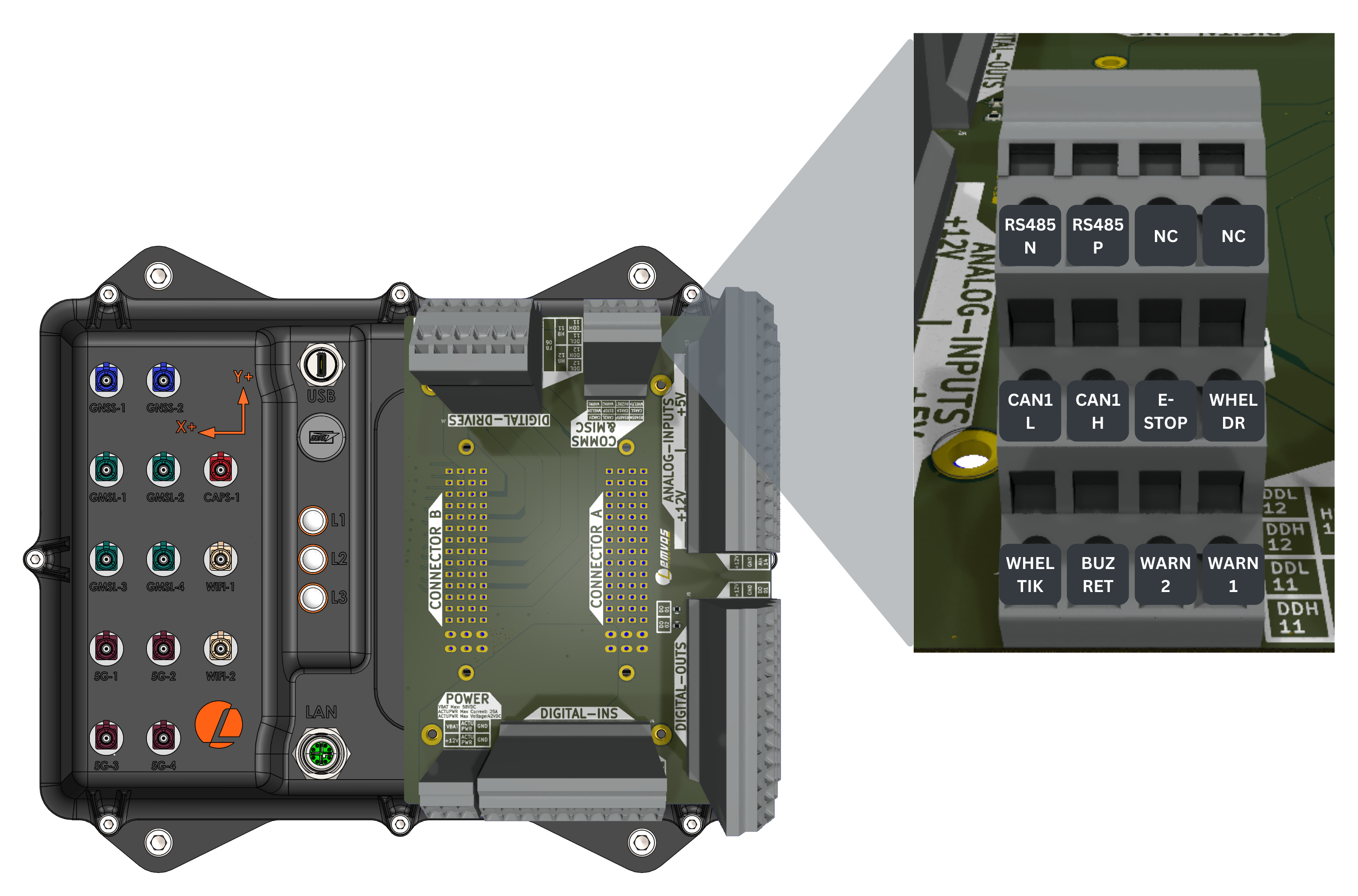
RS485 Specifications
Parameter | Value |
---|---|
Transceiver | THVD1400DR |
Data Rate | 500 kbps |
RS485 Standard | TIA/EIA-485A |
Duplex | Half |
Software
Serial Port
/dev/ttyTHS1
is the serial port used for RS485 communication. RTS
pin is used to control the direction of the transceiver.
RTS
is inverted and active low, RTS
high enables the receiver and RTS
low enables the transmitter.
Example
- Python
- C++
import serial
import serial.rs485
import time
# Configure the serial connections
ser = serial.rs485.RS485(
port='/dev/ttyTHS1',
baudrate=115200, # Set your baud rate
parity=serial.PARITY_NONE,
stopbits=serial.STOPBITS_ONE,
bytesize=serial.EIGHTBITS,
timeout=0.1 # Timeout for read operations
)
ser.rs485_mode = serial.rs485.RS485Settings(
rts_level_for_tx=False,
rts_level_for_rx=True,
)
def send_data(data):
ser.write(data.encode())
print("Sent:", data)
def receive_data():
incoming_data = ser.read(100) # Read up to 100 bytes or until timeout
if incoming_data:
print("Received:", incoming_data.decode()) # Decode bytes to string
if __name__ == '__main__':
try:
while True:
send_data("Hello RS485")
time.sleep(1) # Wait a bit for a response
receive_data()
except Exception as e:
print("An error occurred:", str(e))
finally:
ser.close() # Always close the serial port
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
#include <termios.h>
#include <sys/ioctl.h>
#include <linux/serial.h>
int setup_serial(const char* device, int speed) {
int fd = open(device, O_RDWR | O_NOCTTY | O_SYNC);
if (fd < 0) {
perror("Error opening serial port");
return -1;
}
struct termios tty;
memset(&tty, 0, sizeof tty);
if (tcgetattr(fd, &tty) != 0) {
perror("Error from tcgetattr");
close(fd);
return -1;
}
cfsetospeed(&tty, speed);
cfsetispeed(&tty, speed);
tty.c_cflag |= (CLOCAL | CREAD); // Ignore modem controls, enable reading
tty.c_cflag &= ~CSIZE;
tty.c_cflag |= CS8; // 8-bit characters
tty.c_cflag &= ~PARENB; // No parity bit
tty.c_cflag &= ~CSTOPB; // 1 stop bit
tty.c_cflag &= ~CRTSCTS; // No hardware flow control
tty.c_iflag &= ~(IXON | IXOFF | IXANY); // Turn off s/w flow ctrl
tty.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // Disable canonical mode, echo, and signal chars
tty.c_oflag &= ~OPOST; // Prevent special interpretation of output bytes (e.g., newline chars)
tty.c_cc[VMIN] = 0; // Non-blocking read
tty.c_cc[VTIME] = 20; // 2 seconds read timeout
if (tcsetattr(fd, TCSANOW, &tty) != 0) {
perror("Error from tcsetattr");
close(fd);
return -1;
}
return fd;
}
void set_rts(int fd, int level) {
int status;
ioctl(fd, TIOCMGET, &status); // Get the current status of the modem lines
if (level)
status |= TIOCM_RTS; // Set RTS high
else
status &= ~TIOCM_RTS; // Set RTS low
ioctl(fd, TIOCMSET, &status);
}
void send_data(int fd, const char* data) {
set_rts(fd, 0); // Set RTS low to send
write(fd, data, strlen(data)); // Send data
printf("Sent: %s\n", data);
set_rts(fd, 1); // Set RTS high after sending
}
void receive_data(int fd) {
char buf[100];
int n = read(fd, buf, sizeof(buf)-1);
if (n > 0) {
buf[n] = '\0';
printf("Received: %s\n", buf);
}
}
int main() {
int fd = setup_serial("/dev/ttyTHS1", B9600);
if (fd < 0) {
exit(EXIT_FAILURE);
}
while (1) {
send_data(fd, "Hello RS485");
sleep(1); // Wait a bit for a response
receive_data(fd);
sleep(1);
}
close(fd); // Always close the serial port
return 0;
}
Troubleshooting
- No data is being sent or received: Check the wiring and termination resistors. Check the user permissions and serial port parameters such as baudrate, etc.
- Data is being sent but not received: Check whether the RTS pin is toggled correctly. RTS should be high to recieve data.
- Data is being received but not sent: Check whether the RTS pin is toggled correctly. RTS should be low to send data.
If you are using terminal emulators such as picocom
, toggle the RTS pin manually using the commands offered by the terminal emulator.