Warning Systems
Hardware
Warning system outputs are configured to control standard components such as warning lights and buzzers. Two drivers are allocated for the lighting system, and one driver is designated for a warning buzzer/horn.
Connector Pinout
- Connector
- Development Breakout Board
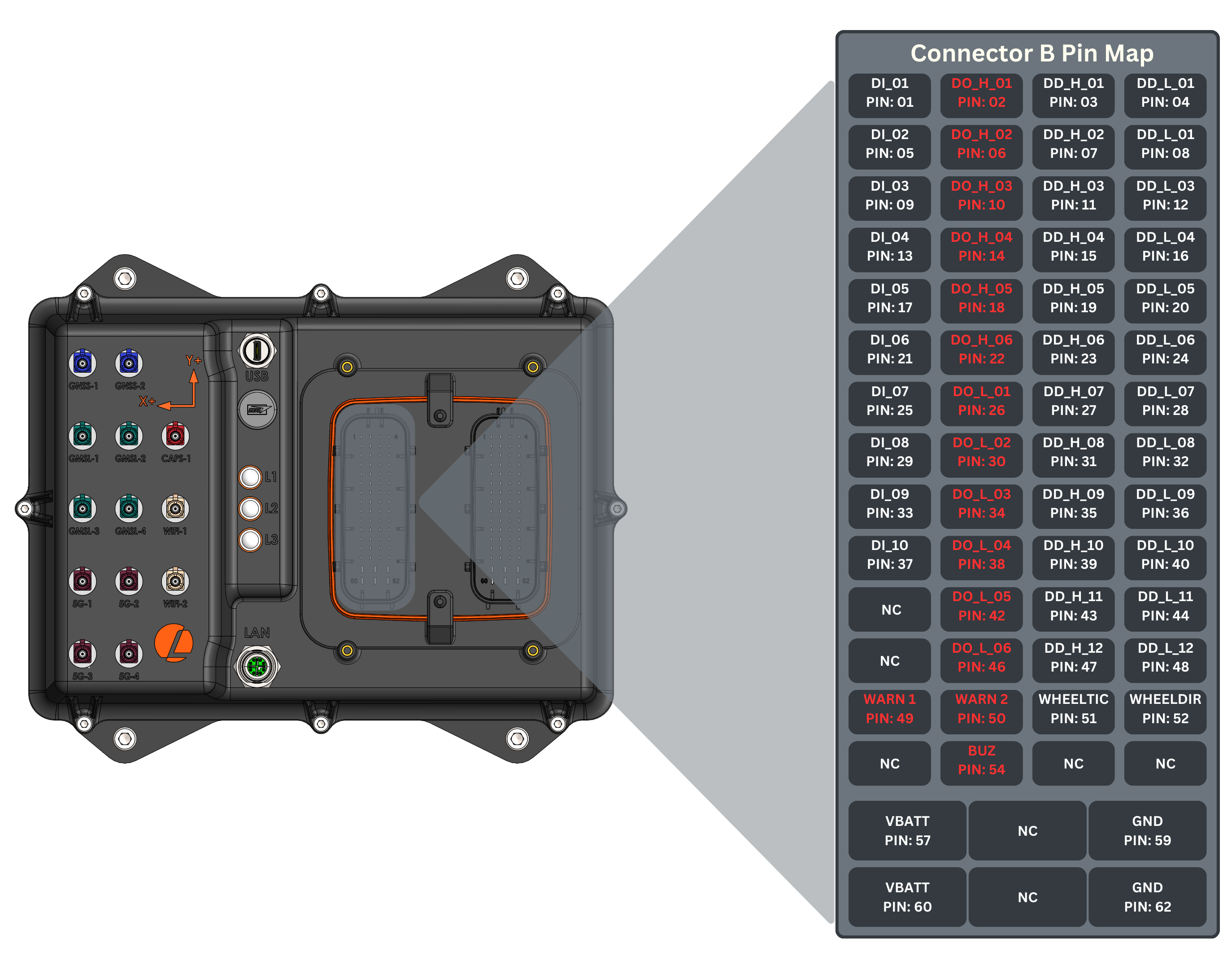
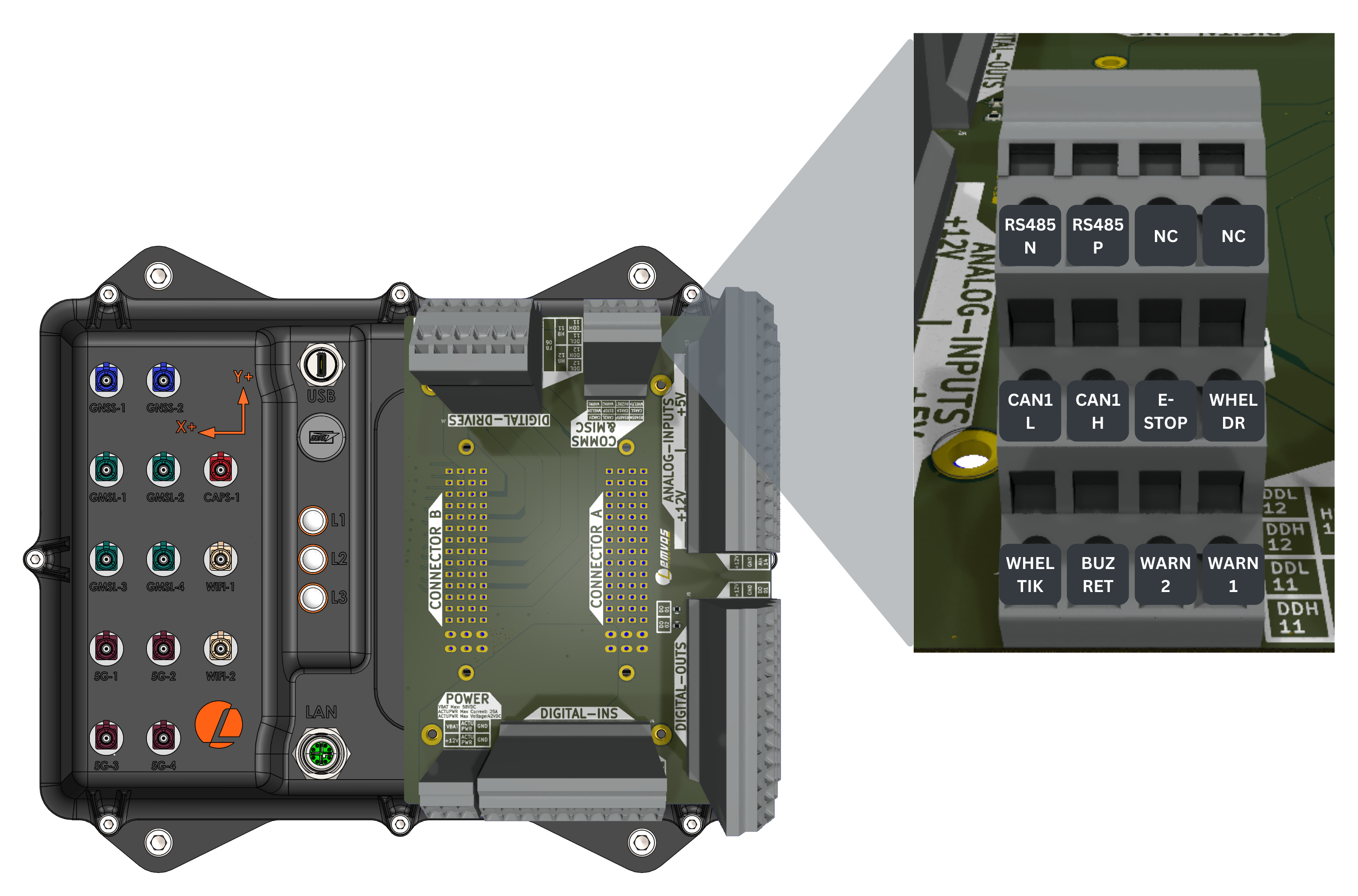
Warning Systems Specifications
Parameter | Value |
---|---|
Onstate | Sinks up to 24V |
Drive Current | 2A |
Controllability | ON/OFF |
Number of Sinks | 3 |
ROS API
Subscribers
Topic | Type | Description |
---|---|---|
/io/warning_systems | automatepro_interfaces/msg/WarningSystems | Publish the desired states for the warning system to this topic. Inputs: warning_system_id : DataType: uint8 (Use ENUM constants defined in msg definiton. Options WarningSystems.WARNING_BUZZER , WarningSystems.WARNING_LIGHT1 , WarningSystems.WARNING_LIGHT_2 ) state : DataType: bool (Use ENUM constants defined in msg definiton. Options WarningSystems.ON , WarningSystems.OFF ) Response Rate: 200 ms |
Example
Example code snippets toggles the Warning Buzzer ON/OFF by publishing to the /io/warning_systems
topic.
- Python
- C++
import rclpy
from rclpy.node import Node
from automatepro_interfaces.msg import WarningSystems
class WarningSystemsPublisher(Node):
def __init__(self):
super().__init__('warning_systems_publisher')
self.publisher_ = self.create_publisher(WarningSystems, '/io/warning_system_out', 10)
self.timer = self.create_timer(1.0, self.timer_callback) # 1s
self.state = False
def timer_callback(self):
msg = WarningSystems()
msg.warning_system_id = WarningSystems.WARNING_BUZZER
msg.state = self.state
self.publisher_.publish(msg)
self.get_logger().info(
'Publishing WarningSystems: warning_system_id=%d, state=%d' %
(msg.warning_system_id, msg.state))
self.state = not self.state # Toggle state
def main(args=None):
rclpy.init(args=args)
node = WarningSystemsPublisher()
rclpy.spin(node)
node.destroy_node()
rclpy.shutdown()
if __name__ == '__main__':
main()
Run the node using the following command:
ros2 run automatepro_python_tutorials warning_system_out_node
#include <rclcpp/rclcpp.hpp>
#include <automatepro_interfaces/msg/warning_systems.hpp>
class WarningSystemsPublisher : public rclcpp::Node
{
public:
WarningSystemsPublisher()
: Node("warning_systems_publisher"), state_(false)
{
publisher_ = this->create_publisher<automatepro_interfaces::msg::WarningSystems>("/io/warning_system_out", 10);
timer_ = this->create_wall_timer(
std::chrono::seconds(1),
std::bind(&WarningSystemsPublisher::timer_callback, this));
}
private:
void timer_callback()
{
auto msg = automatepro_interfaces::msg::WarningSystems();
msg.warning_system_id = automatepro_interfaces::msg::WarningSystems::WARNING_BUZZER; // Change to WARNING_LIGHT1 or WARNING_LIGHT2 as needed
msg.state = state_;
publisher_->publish(msg);
RCLCPP_INFO(this->get_logger(), "Publishing WarningSystems: warning_system_id=%d, state=%d",
msg.warning_system_id, msg.state);
state_ = !state_; // Toggle state
}
rclcpp::Publisher<automatepro_interfaces::msg::WarningSystems>::SharedPtr publisher_;
rclcpp::TimerBase::SharedPtr timer_;
bool state_;
};
int main(int argc, char *argv[])
{
rclcpp::init(argc, argv);
rclcpp::spin(std::make_shared<WarningSystemsPublisher>());
rclcpp::shutdown();
return 0;
}
Run the node using the following command:
ros2 run automatepro_cpp_tutorials warning_system_out_node